borea
Change the text and make it your own. Click here to begin editing.
Boujee Gras Vibes Cropped Fleece Pullover
Change the text and make it your own. Click here to begin editing.
Boujee Gras Vibes Tee
Change the text and make it your own. Click here to begin editing.
Boujee on Bourbon Hoodie
Change the text and make it your own. Click here to begin editing.
I'm a product
Change the text and make it your own. Click here to begin editing.
I'm a product
Change the text and make it your own. Click here to begin editing.
I'm a product
Change the text and make it your own. Click here to begin editing.
I'm a product
Change the text and make it your own. Click here to begin editing.
It's A Boujee Kind Of Day Spiral Notebook
Change the text and make it your own. Click here to begin editing.
It's Boujee Gras Baaeba Hoodie
Change the text and make it your own. Click here to begin editing.
It's Boujee Gras Baaeba Tumbler
Change the text and make it your own. Click here to begin editing.
Let the Mardi Swag Roll Long Sleeve Tee
Change the text and make it your own. Click here to begin editing.
Mardi Gras Squad Hoodie
Change the text and make it your own. Click here to begin editing.
Pour Me Something Boujee Hoodie
Change the text and make it your own. Click here to begin editing.
borea2
Change the text and make it your own. Click here to begin editing.
Boujee Gras Vibes Hoodie
Change the text and make it your own. Click here to begin editing.
Boujee on Bourbon
Change the text and make it your own. Click here to begin editing.
Boujee on Bourbon Long Sleeve Tee
Change the text and make it your own. Click here to begin editing.
I'm a product
Change the text and make it your own. Click here to begin editing.
I'm a product
Change the text and make it your own. Click here to begin editing.
I'm a product
Change the text and make it your own. Click here to begin editing.
It's A Boujee Kind of Day
Change the text and make it your own. Click here to begin editing.
It's a Boujee Kind of Day Tumbler
Change the text and make it your own. Click here to begin editing.
It's Boujee Gras Baaeba Long Sleeve Tee
Change the text and make it your own. Click here to begin editing.
Let the Mardi Swag Roll Crewneck Sweatshirt
Change the text and make it your own. Click here to begin editing.
Let the Mardi Swag Roll Tee
Change the text and make it your own. Click here to begin editing.
Mardi Gras Squad Long Sleeve Tee
Change the text and make it your own. Click here to begin editing.
Pour Me Something Boujee Long Sleeve Tee
Change the text and make it your own. Click here to begin editing.
Boujee Gras Vibes Crewneck Sweatshirt
Change the text and make it your own. Click here to begin editing.
Boujee Gras Vibes Long Sleeve Tee
Change the text and make it your own. Click here to begin editing.
Boujee on Bourbon Crewneck Sweatshirt
Change the text and make it your own. Click here to begin editing.
btedadsra
Change the text and make it your own. Click here to begin editing.
I'm a product
Change the text and make it your own. Click here to begin editing.
I'm a product
Change the text and make it your own. Click here to begin editing.
I'm a product
Change the text and make it your own. Click here to begin editing.
It's A Boujee Kind of Day Hoodie
Change the text and make it your own. Click here to begin editing.
It's Boujee Gras Baaeba Crewneck Sweatshirt
Change the text and make it your own. Click here to begin editing.
It's Boujee Gras Baaeba Tee
Change the text and make it your own. Click here to begin editing.
Let the Mardi Swag Roll Hoodie
Change the text and make it your own. Click here to begin editing.
Mardi Gras Squad Crewneck Sweatshirt
Change the text and make it your own. Click here to begin editing.
Mardi Gras Squad Tee
Change the text and make it your own. Click here to begin editing.
Pour Me Something Boujee Tee
Change the text and make it your own. Click here to begin editing.
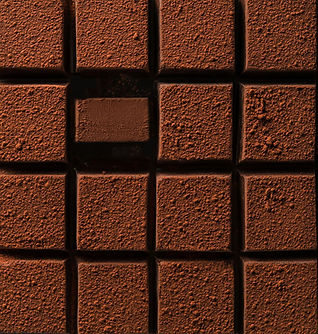
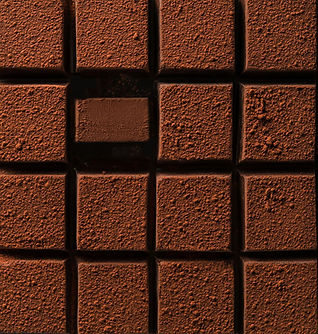
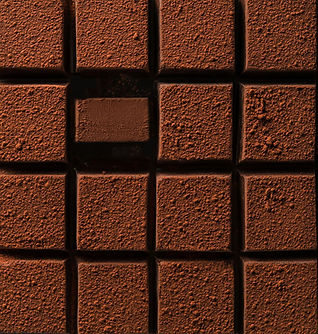
<script>
document.addEventListener('DOMContentLoaded', function() {
// Find the specific empty state container
const container = document.querySelector('[data-hook="empty-state-container"]');
// Check if container exists and contains "Check back soon" text
if (container && container.textContent.includes('Check back soon')) {
// 1. Apply white background to container
container.style.backgroundColor = 'white';
// 2. Apply black color to title element
const title = container.querySelector('[data-hook="empty-states__title"]');
if (title) {
title.style.color = 'black';
// Also target the nested div if needed
const titleInner = title.querySelector('.cJscj1');
if (titleInner) titleInner.style.color = 'black';
}
// 3. Apply black color to description
const description = container.querySelector('.cTdFFO.blog-post-homepage-description-color');
if (description) {
description.style.color = 'black';
// Also target the nested div if needed
const descInner = description.querySelector('.clf9mN');
if (descInner) descInner.style.color = 'black';
}
}
});
</script>
If you're already log in, presss here
import wixData from 'wix-data';
$w.onReady(function () {
$w("#ddata1").onSubmitSuccess(() => {
const formFieldValues = $w("#ddata1").getFieldValues();
console.log("Form submitted successfully with the following values: ", formFieldValues);
// Insert data into the original collection if needed
wixData.insert('OriginalCollectionName', formFieldValues)
.then((results) => {
console.log('Data inserted successfully into the original collection', results);
})
.catch((err) => {
console.error('Error inserting data into the original collection', err);
});
// Prepare the data for insertion into the second collection with different field names
const toInsertInMyTestFormCopy = {
email: formFieldValues.email_091e, // Assuming 'emailField' is the field name in MYTESTFORMCOPY
firstName: formFieldValues.first_name_4025, // 'firstNameField' in MYTESTFORMCOPY
fixedPrice: JSON.stringify(formFieldValues.fixed_price_cc6d), // 'pricingDetails' in MYTESTFORMCOPY
imageGallery: JSON.stringify(formFieldValues.gallery), // 'imageGallery' in MYTESTFORMCOPY
lastName: formFieldValues.last_name_3bca, // 'lastNameField' in MYTESTFORMCOPY
nameToBeDisplayed: formFieldValues.member_name, // 'username' in MYTESTFORMCOPY
multiLineAddress: JSON.stringify(formFieldValues.multi_line_address_b2cc) // 'address' in MYTESTFORMCOPY
};
// Insert data into 'MYTESTFORMCOPY'
wixData.insert('MYTESTFORMCOPY', toInsertInMyTestFormCopy)
.then((results) => {
console.log('Data inserted successfully into MYTESTFORMCOPY', results);
})
.catch((err) => {
console.error('Error inserting data into MYTESTFORMCOPY', err);
});
});
});
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta name="viewport" content="width=device-width, initial-scale=1.0" />
<title>اختر قالب التهنئة الخاص بك</title>
<style>
body {
font-family: Arial, sans-serif;
text-align: center;
background-color: #f4f4f4;
padding: 20px;
}
.container {
max-width: 800px;
margin: 0 auto;
background: #fff;
padding: 20px;
border-radius: 10px;
box-shadow: 0 0 10px rgba(0, 0, 0, 0.1);
}
.image-list {
display: flex;
flex-wrap: wrap;
justify-content: center;
gap: 10px;
margin-bottom: 20px;
}
.image-list img {
width: 100px;
height: 100px;
object-fit: cover;
border: 2px solid #ccc;
border-radius: 5px;
cursor: pointer;
}
.image-list img.selected {
border-color: #28a745;
}
canvas {
border: 1px solid #ccc;
margin-top: 20px;
max-width: 100%;
}
input[type="text"],
input[type="number"],
button,
select {
margin: 10px 0;
padding: 10px;
width: 100%;
border: 1px solid #ccc;
border-radius: 5px;
}
.controls, .coords {
display: flex;
gap: 10px;
margin-bottom: 20px;
flex-wrap: wrap;
}
.controls select, .coords input {
width: auto;
flex: 1;
}
button {
background-color: #28a745;
color: white;
cursor: pointer;
}
button:hover {
background-color: #218838;
}
</style>
</head>
<body>
<div class="container">
<h1>اختر قالب التهنئة الخاص بك</h1>
<div class="image-list" id="imageList">
<!-- Images will be dynamically added here -->
</div>
<div class="controls">
<input type="text" id="textInput" placeholder="Enter your text here..." />
<select id="colorPicker">
<option value="white">White</option>
<option value="black">Black</option>
<option value="red">Red</option>
<option value="blue">Blue</option>
<option value="green">Green</option>
<option value="yellow">Yellow</option>
</select>
<select id="fontSizePicker">
<option value="40">40px</option>
<option value="50">50px</option>
<option value="60">60px</option>
<option value="70">70px</option>
<option value="80">80px</option>
<option value="90">90px</option>
<option value="100">100px</option>
<option value="120">120px</option>
<option value="140">140px</option>
<option value="160">160px</option>
</select>
</div>
<div class="coords">
<input type="number" id="xInput" placeholder="X coordinate" />
<input type="number" id="yInput" placeholder="Y coordinate" />
</div>
<button id="downloadBtn">Download Image</button>
<canvas id="canvas"></canvas>
</div>
<script>
const imageList = document.getElementById('imageList');
const textInput = document.getElementById('textInput');
const colorPicker = document.getElementById('colorPicker');
const fontSizePicker = document.getElementById('fontSizePicker');
const xInput = document.getElementById('xInput');
const yInput = document.getElementById('yInput');
const canvas = document.getElementById('canvas');
const downloadBtn = document.getElementById('downloadBtn');
const ctx = canvas.getContext('2d');
let selectedImage = null;
let text = "";
let textColor = "Black"; // Default text color
let fontSize = 60; // Default font size
// Global coordinates for the text
let textX = 0;
let textY = 0;
// List of image URLs
const imageUrls = [
"https://static.wixstatic.com/media/458db9_7b22f40e68484f8bb55a7677eb9ca482~mv2.jpg",
"https://static.wixstatic.com/media/458db9_3056eac77b194c04b99d229f954f12a1~mv2.jpg",
"https://static.wixstatic.com/media/458db9_3056eac77b194c04b99d229f954f12a1~mv2.jpg"
];
// Load images into the list
imageUrls.forEach((url, index) => {
const imgElement = document.createElement('img');
imgElement.src = url;
imgElement.alt = `Image ${index + 1}`;
imgElement.addEventListener('click', () => selectImage(url, imgElement));
imageList.appendChild(imgElement);
});
// Handle image selection
function selectImage(url, imgElement) {
// Remove 'selected' class from all images
document.querySelectorAll('.image-list img').forEach(img => img.classList.remove('selected'));
// Add 'selected' class to the clicked image
imgElement.classList.add('selected');
// Load the selected image onto the canvas
const img = new Image();
img.crossOrigin = "anonymous";
img.onload = () => {
selectedImage = img;
canvas.width = img.width;
canvas.height = img.height;
// Set default coordinates: center horizontally, 10% from bottom
textX = canvas.width / 2;
textY = canvas.height * 0.8;
// Update coordinate inputs with default values
xInput.value = Math.round(textX);
yInput.value = Math.round(textY);
drawImageAndText();
};
img.src = url;
}
// Handle text input
textInput.addEventListener('input', () => {
text = textInput.value;
drawImageAndText();
});
// Handle color picker change
colorPicker.addEventListener('change', () => {
textColor = colorPicker.value;
drawImageAndText();
});
// Handle font size picker change
fontSizePicker.addEventListener('change', () => {
fontSize = parseInt(fontSizePicker.value);
drawImageAndText();
});
// Handle coordinate input changes
xInput.addEventListener('input', () => {
textX = parseInt(xInput.value) || 0;
drawImageAndText();
});
yInput.addEventListener('input', () => {
textY = parseInt(yInput.value) || 0;
drawImageAndText();
});
// Draw image and text on canvas using global coordinates
function drawImageAndText() {
if (!selectedImage) return;
ctx.clearRect(0, 0, canvas.width, canvas.height);
ctx.drawImage(selectedImage, 0, 0, canvas.width, canvas.height);
if (text) {
ctx.font = `${fontSize}px Arial`;
ctx.fillStyle = textColor;
ctx.strokeStyle = "black";
ctx.lineWidth = 2;
ctx.textAlign = "center";
ctx.textBaseline = "top";
ctx.strokeText(text, textX, textY);
ctx.fillText(text, textX, textY);
}
}
// Handle download button click
downloadBtn.addEventListener('click', () => {
if (selectedImage) {
const imageUrl = canvas.toDataURL('image/png');
const newWindow = window.open();
newWindow.document.write(`<img src="${imageUrl}" alt="Custom Image" style="max-width: 100%; height: auto;" />`);
newWindow.document.close();
} else {
alert("Please select an image first.");
}
});
</script>
</body>
</html>
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Image Text Overlay Tool</title>
<style>
body {
font-family: Arial, sans-serif;
text-align: center;
background-color: #f4f4f4;
padding: 20px;
}
.container {
max-width: 800px;
margin: 0 auto;
background: #fff;
padding: 20px;
border-radius: 10px;
box-shadow: 0 0 10px rgba(0, 0, 0, 0.1);
}
.image-list {
display: flex;
justify-content: center;
gap: 10px;
margin-bottom: 20px;
}
.image-list img {
width: 100px;
height: 100px;
object-fit: cover;
border: 2px solid #ccc;
border-radius: 5px;
cursor: pointer;
}
.image-list img.selected {
border-color: #28a745;
}
canvas {
border: 1px solid #ccc;
margin-top: 20px;
max-width: 100%;
cursor: move;
}
input[type="text"], button {
margin: 10px 0;
padding: 10px;
width: 100%;
border: 1px solid #ccc;
border-radius: 5px;
}
button {
background-color: #28a745;
color: white;
cursor: pointer;
}
button:hover {
background-color: #218838;
}
</style>
</head>
<body>
<div class="container">
<h1>Image Text Overlay Tool</h1>
<div class="image-list" id="imageList">
<!-- Images will be dynamically added here -->
</div>
<input type="text" id="textInput" placeholder="Enter your text here..." />
<button id="downloadBtn">Download Image</button>
<canvas id="canvas"></canvas>
</div>
<script>
const imageList = document.getElementById('imageList');
const textInput = document.getElementById('textInput');
const canvas = document.getElementById('canvas');
const downloadBtn = document.getElementById('downloadBtn');
const ctx = canvas.getContext('2d');
let selectedImage = null;
let text = "";
let textX = 100; // Initial X position of the text
let textY = 100; // Initial Y position of the text
let isDragging = false;
// List of image URLs
const imageUrls = [
"https://static.wixstatic.com/media/c21d41_4baa8c7d84b142e1afbbd912a3fdf943~mv2.png",
"https://static.wixstatic.com/media/c21d41_30f0e9be65ba439f807344cf50723a87~mv2.png"
];
// Load images into the list
imageUrls.forEach((url, index) => {
const imgElement = document.createElement('img');
imgElement.src = url;
imgElement.alt = `Image ${index + 1}`;
imgElement.addEventListener('click', () => selectImage(url, imgElement));
imageList.appendChild(imgElement);
});
// Handle image selection
function selectImage(url, imgElement) {
// Remove 'selected' class from all images
document.querySelectorAll('.image-list img').forEach(img => img.classList.remove('selected'));
// Add 'selected' class to the clicked image
imgElement.classList.add('selected');
// Load the selected image onto the canvas
const img = new Image();
img.crossOrigin = "anonymous"; // Allow cross-origin images
img.onload = () => {
selectedImage = img;
canvas.width = img.width;
canvas.height = img.height;
drawImageAndText();
};
img.src = url;
}
// Handle text input
textInput.addEventListener('input', () => {
text = textInput.value;
drawImageAndText();
});
// Draw image and text on canvas
function drawImageAndText() {
ctx.clearRect(0, 0, canvas.width, canvas.height);
ctx.drawImage(selectedImage, 0, 0, canvas.width, canvas.height);
if (text) {
ctx.font = "40px Arial";
ctx.fillStyle = "white";
ctx.strokeStyle = "black";
ctx.lineWidth = 2;
ctx.textAlign = "center";
ctx.textBaseline = "top";
// Draw text at the current position
ctx.strokeText(text, textX, textY);
ctx.fillText(text, textX, textY);
}
}
// Handle mouse events for dragging text
canvas.addEventListener('mousedown', (e) => {
const rect = canvas.getBoundingClientRect();
const mouseX = e.clientX - rect.left;
const mouseY = e.clientY - rect.top;
// Check if the mouse is near the text
if (
mouseX >= textX - 50 && mouseX <= textX + 50 &&
mouseY >= textY - 20 && mouseY <= textY + 20
) {
isDragging = true;
}
});
canvas.addEventListener('mousemove', (e) => {
if (isDragging) {
const rect = canvas.getBoundingClientRect();
textX = e.clientX - rect.left;
textY = e.clientY - rect.top;
drawImageAndText();
}
});
canvas.addEventListener('mouseup', () => {
isDragging = false;
});
canvas.addEventListener('mouseleave', () => {
isDragging = false;
});
// Handle download button click
downloadBtn.addEventListener('click', () => {
if (selectedImage) {
// Convert canvas to data URL
const imageUrl = canvas.toDataURL('image/png');
// Open the image in a new tab
const newWindow = window.open();
newWindow.document.write(`<img src="${imageUrl}" alt="Custom Image" style="max-width: 100%; height: auto;" />`);
newWindow.document.close();
} else {
alert("Please select an image first.");
}
});
</script>
</body>
</html>
import { orders } from "wix-pricing-plans-frontend";
import wixLocationFrontend from 'wix-location-frontend';
$w.onReady(() => {
orders
.listCurrentMemberOrders()
.then((ordersList) => {
console.log(ordersList)
// Check if any active orders match the specified plans
const hasIndividual = ordersList.some(order => order.planName === "Individual" && order.status === "ACTIVE");
const hasCompany = ordersList.some(order => order.planName === "Company" && order.status === "ACTIVE");
// Array of URLs for each button
const urls = [
'/path-for-item1',
'/path-for-item2',
'/path-for-item3',
'/path-for-item4',
'/path-for-item5',
'/path-for-item6',
'/path-for-item7',
'/path-for-item8',
'/path-for-item9'
];
// Default path if no specific plan is active
const defaultPath = '/plans-pricing';
// Set the URL for each button based on whether the user has an active plan
$w("#repeater1").forEachItem(($item, itemData, index) => {
let path = (hasIndividual || hasCompany) ? urls[index] : defaultPath;
$item("#button24").onClick(() => {
console.log(path)
wixLocationFrontend.to(path);
});
});
})
.catch((error) => {
console.error("Error retrieving orders:", error);
});
});